CODING TOUR
Welcome to the Dan Zen Museum Coding Tour. As a reward for taking the tour, we will tell you that one word is out of place. If you combine this word with out-of-place words from the other tours you will have a secret message. Dan Zen encourages you to slow down a little - relax, enjoy and fully experience the museum. And now, without further meta, on with the tour!
GUIDE
THOUGHTS ON CODINGCODE EXAMPLE
CODING BASICS
DAN ZEN CODING
CONCLUSION
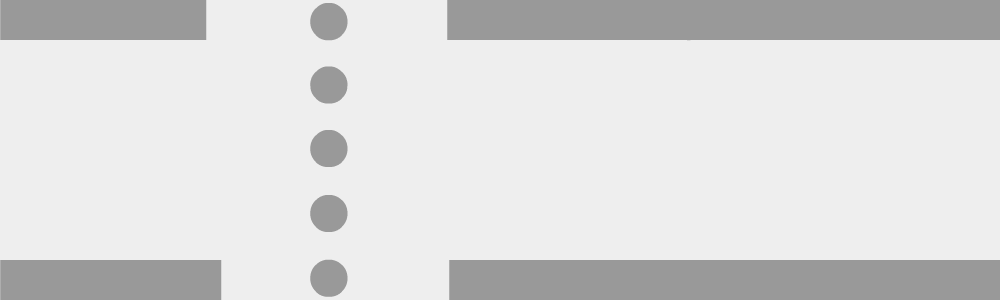
Coding, or computer programming, is how Dan Zen builds his digital creations. Coding sets up and enforces the rules of a game, the boundaries of an environment, applies the logic to artificial intelligence and the dynamics to generative art. The first stages for creating a project including ideation and asset creation (graphics, sound, etc.) are explored in the CREATIVITY TOUR. Now let's see what Dan Zen has to say about bringing the assets to life with code.
"Computer coding is how interactive media is created. Without code, you would have just text, audio, video, animation and sound as we have had in traditional media like books, magazines, the radio, film and TV.
Screenshot of a Dan Zen interactive Flash Example used for teaching code.
In life, we have rules that form and govern environments. These range from the basics - the laws of physics to the specifics - such as the nuances of social communication. Coding is a way to apply these rules with a computer. With code we can model life."
Our next room shows an example of code.
Dan Zen explains, "An example of modeling life can be found in the Moustache Mysteries series. You ask computer characters about certain topics and they may give you clues. The code determines which clues will be given. Here is the simplified code for this scenario.
Character Drawings by Greg Rennick - backgrounds and production by Dan Zen
Clues that the characters know are put in a list as are the clue topics. Each clue has a statement, a sensitivity out of 10 and the status of whether the character has told you the clue already. Lists in code (Arrays) are indexed starting at 0 instead of 1. In the topics list, the numbers mean that diamonds relate to the first clue. Paintings relate to the first and second clue and beatnik to the third clue.
clues = [
When you use the mystery game interface to ask a character about a topic (say, painting), the code gets the list of the clues the character has about the topic.
topic = "painting"
This gives the list of [0,1] and stores it in a variable (holder) called relevantClues. The code then picks a random clue from the list.
clue = relevantClues[Math.floor( Math.random() * relevantClues.length )]
Math.random() makes a random number between 0 and 1 - so it could be .347 and the code multiplies it by the length of the list which is 2 and gets .694. Math.floor() removes decimals so the number becomes 0. If the random number was .801 then multiplying by 2 would be 1.602 and when the code floors this it is 1. With this code, if the length is 2 we will always have 0 or 1. If the length is 20 we would always get a number from 0-19. The code then gets the clue at this random index. If the index is 0 then the clue is the first clue in clues, etc.
This code looks a little complex, and it is a common task, so we can make a custom function to do the code and then reuse it. If we made that custom function and called it getRandomElement, then we could say:
clue = getRandomElement(relevantClues)
Let's imagine that the clue the code picks is the second clue:
Once we have the clue, the code will decide whether the character tells the clue. We get a random number out of 10 and test to see if it is greater than the sensitivity of the clue.
if (Math.random()*10 > clue[1]) {
If the random number is .433 then multiplying by 10 gives 4.33. clue[1] gets the second element of the clue which is the sensitivity, in this case, 3. So since 4.33 is bigger than 3 the character will tell the clue - that is as long as they have not already told the clue.
if (clue[2] == true) {
To make the mystery more realistic, code was added to provide the character with a secret keeping capability out of 10. If the clue has a sensitivity of greater than 5 then the code gets another random number and if that number is greater than the secret keeping capability then the character tells the clue. But wait... code was also added to make it so the clue would not be told if certain other characters were in the room. And wait... it turns out that the clue can be passed from character to character and as it gets passed, it may get slightly muddled. Serge said that Professor Y said that painting is of a cow.
If in the end, the character does tell the clue then we must record that fact - tell the clue in the clues list at index 1 that its status (index 2) should be set to true because we have told the clue.
clues[1][2] = true
This is one example of how code works. There are many other things that code can do such as animate a ball across the screen and find out if it is hitting a wall or if you have pressed on the ball. Code could then make the ball disappear or grow bigger or play a sound. Code could make a new page show up and fill in text that a friend has provided from across the world. Code can determine which key is being pressed, it can tell if you have swiped or tilted your mobile device."
Let's take a look at the parts of coding that let you do this.
There are different programming languages such as C, Java, JavaScript, ActionScript, PHP, etc. They all have the same basics but may have different syntax. Syntax is the way the code is written. Launguages can be broken down into A) how logic is applied and B) how assets are managed, as outlined below.
A Typically, logic is applied the same way in computer languages. Variables are used to store things and conditionals are used to test the variables. There are also efficiencies such as functions, loops and arrays that help organize the flow of the logic.
A variable is a holder, for instance:
name="Dan" // or
Now, anytime we use name we are PRINCIPLES really using "Dan" and when we use x, we are really using 10. The variable can change (hence variable) so we can set the variable to 20 by saying x=20. A variable is like memory.
A conditional is an if statement:
if (name=="Dan") {
A function is a block of code that does something:
function doSomething() {
The block of code could be one statement or many statements. You can call the function any time: doSomething() at which point it runs the block of code. You can call a function more than once. The round brackets after the function name is to pass extra information (parameters) to the function.
A loop lets you repeat a block of code multiple times:
for (i=0; i<100; i++) {
This might be handy to make 100 monsters.
An array is like a list of variables:
myGreetings = ["hello", "salut", "ciao", "aloha"]
You can then access any element of the array using the index number myGreetings[0] would be "hello".
The computer will read each statement (usually a line of code) and generally do them in order unless you are calling a function, or doing a loop, in which case it does the code in the function or loop and then proceeds to the code after the function or the loop.
B Aside from the common ways to handle logic in coding, there is how to handle assets. Assets are the things that the user sees or hears - buttons, pictures, animations, sounds... Currently, the most popular way to handle assets in code is with classes from which objects are made and so most current languages are called Object Oriented languages and hence Object Oriented Programming or OOP. Objects have properties and methods that can be changed or called when events happen.
A class is a template from which objects are made.
An object is an independent instance of the class. You might think of an object as a noun - a person, place or thing.
A property is a variable that belongs to the object. You might think of a property as an adjective. For instance, a Sound object has duration and volume properties.
A method is a function that belongs to the object. You might think of a method as a verb. Something that the object does or is done to the object. For instance, a Sound object has play() and stop() methods.
An event is something that happens such as the user clicking or pressing a key. A timer has an event. There is an event when something is finished loading, etc. There are at least a couple dozen events we use all the time to make the game or application proceed.
// make object from class
The objects made from a class are independent so they have their own property values and the methods act only on the object on which you call the method. You can also apply events to an object that trigger a method. For instance, you could add an event to the Sound object that calls a method when the sound is complete. This method may make another object, change properties of an object, call another method or set another event, etc. and so the code continues. What about Dan Zen code?
Dan Zen won the Canadian New Media Awards Programmer of the Year in 2002 for his work on the Dan Zen site of games, gadgets and communities. Below are some of the images that accompanied his entry. Motogami was made afterwards and is included because it was sort of cool using code as the banner for the site.
Dan Zen first started coding in 1982 in Engineering at McMaster University in Basic on a Vax system and Sharp hand held calculators. He then went on to code in Fortran and the AskSam database language to augment his patent work in the early 90's.
His early multimedia work was in Macromedia Director which had a language called Lingo. He programmed for the Web in Perl and flatfiles for data until he switched over to PHP and MySQL sometime around 2000. At the same time, he switched to Flash for his multimedia with his Opartica application. The interactive features are supported on the backend by PHP and MySQL. He proceeded to make Web apps and games in Flash like RichDeck, Tilator, Zen Motto, the MotoGami games, etc.
Dan Zen has made about 40 Flash apps since and still uses Flash for his recent games Touchy, Tilty, Wavy, Swoodle, Hipster, Hangy, Gobstop, Trippy and Droner. He has also made a number of sites in HTML, CSS and JavaScript but these are primarily menu based like the Dark menu and the Dan Zen Museum - the steps of which are advanced HTML 5 work using CreateJS to mimic Flash.
Dan Zen is a strong advocate for Flash with many posts encouraging developers and the interactive industry to keep an open mind despite their allegence to open source. He believes that closed source often provides a more forward thinking roadmap and it is not good for our culture to do things like take away plugins from browsers.
Here are some statistics for Dan Zen features of the early 2010's in terms of lines of code:
THOUGHTS ON CODING
GUIDE
THOUGHTS ON CODING
CODE EXAMPLE
CODING BASICS
DAN ZEN CODING
CONCLUSION
CODE EXAMPLE
["the diamond is behind the painting",9,false],
["the painting is ugly",3,false],
["a beatnik is here",3,false], etc. ]
topics = [diamonds:[0], painting:[0,1], beatnik:[2], etc.]
relevantClues = topics[topic]
// better? (slash slash means a comment)
["the painting is ugly",3,false]
// tell clue
} else {
// do not tell clue
}
// do not tell clue
// say they already told you!
} else {
//tell the clue
}
GUIDE
THOUGHTS ON CODING
CODE EXAMPLE
CODING BASICS
DAN ZEN CODING
CONCLUSION
CODING BASICS
x=10
// do this
}
//or
if (x < 10) {
// do this
} else {
// do that
}
//here is the block of code
}
// to call the function you use:
doSomething()
//do this 100 times
}
trace( myGreetings[0] )
// outputs hello
monster = new Monster();
// set some properties
monster.x = 200
monster.y = 300
monster.color = 0x0f0 // green
// add an event
monster.addEventListener( MouseEvent.CLICK, tickle )
// event function
function tickle() {
// call a method
monster.growl();
monster.scaleX = 2;
monster.scaleY = 2;
}
GUIDE
THOUGHTS ON CODING
CODE EXAMPLE
CODING BASICS
DAN ZEN CODING
CONCLUSION
DAN ZEN CODING
APPLICATION LINES Hipster 1,700 Hangy 2,000 Tilty 600 Swoodle 550 Droner 1,500 Gobstop 1,000 Custom Classes 34,500
Taking an average project to be 1000 lines of code over approximately 75 projects (not including Websites) and adding the custom classes, Dan Zen has roughly 100,000 lines of code.
Here is the main Gobstop code.
GUIDE
THOUGHTS ON CODINGCODE EXAMPLE
CODING BASICS
DAN ZEN CODING
CONCLUSION

CONCLUSION
Dan Zen thinks that coding is the most wonderful puzzle with an outcome that can be useful for society - to encourage them to create, learn, communicate and play. He also sees logical people modeling life and hopes that one day, they will realize what they are - philosophers, after all, philosophy is a modeling of life. To this end, Dan Zen started the Philosophy of Nodism which you can explore in the NODISM TOUR.